Beyond Models: How Vector Databases Are Transforming AI Applications
2025-04-04
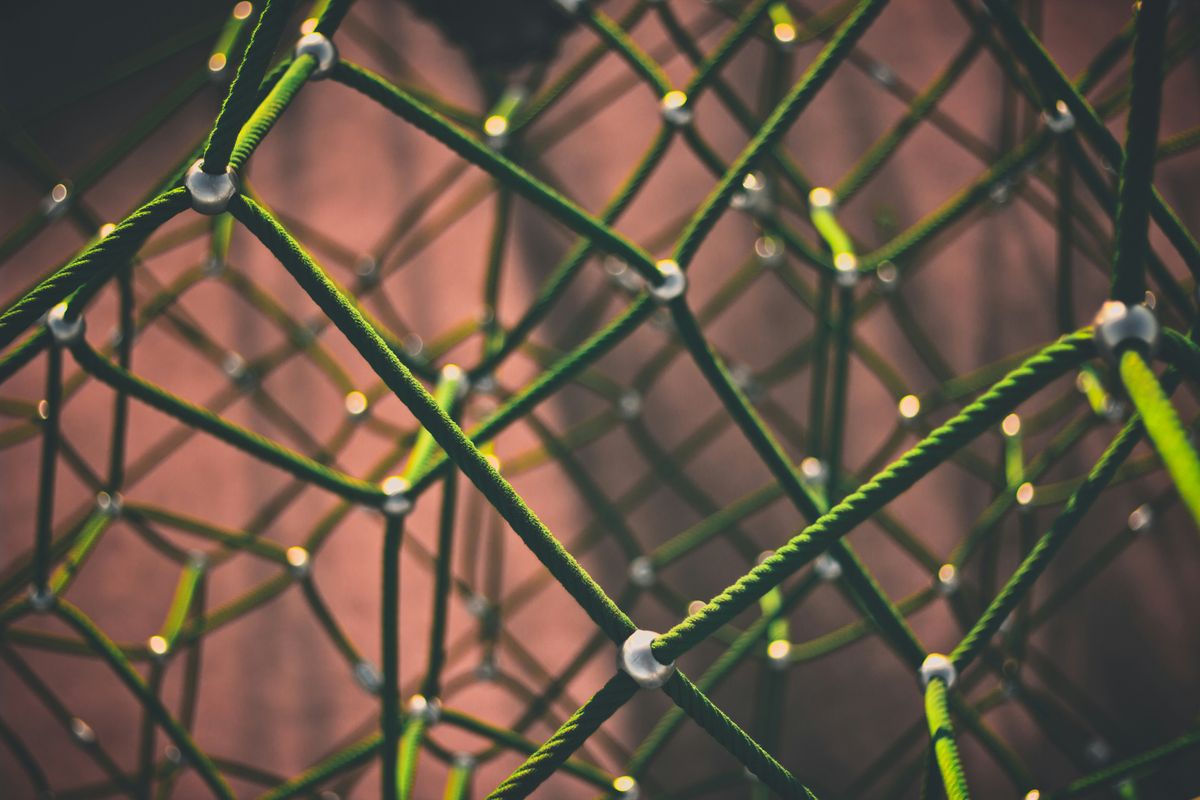
While the spotlight often shines on large language models and neural networks, the infrastructure that powers production AI applications deserves equal attention. Vector databases have quietly become one of the most transformative technologies in the AI developer's toolkit, enabling everything from semantic search to recommendation engines. As applications demand more contextual awareness and similarity-based intelligence, understanding these specialized databases is no longer optional—it's essential for any developer working at the intersection of AI and software engineering.
What Makes Vector Databases Special?
Traditional databases excel at exact matches and range queries, but struggle with the fundamental question that drives modern AI applications: "What's similar to this?" Vector databases fill this gap by storing and efficiently querying high-dimensional vector embeddings—numerical representations of data that capture semantic meaning.
When you convert text, images, audio, or any data into embeddings using models like OpenAI's text-embedding-ada-002 or CLIP, these vectors preserve similarity relationships. Words with similar meanings cluster together in vector space. Images with similar content appear near each other. But storing and searching these vectors efficiently requires specialized infrastructure.
# Converting text to embeddings with OpenAI
import openai
response = openai.Embedding.create(
input="Vector databases are essential for AI applications",
model="text-embedding-ada-002"
)
embedding = response['data'][0]['embedding']
# This embedding is a 1,536-dimensional vector that represents the semantic meaning
The Anatomy of a Vector Database
Vector databases differ from traditional databases in several critical ways:
-
Approximate Nearest Neighbor (ANN) Algorithms: Instead of exact matches, they use algorithms like HNSW, IVF, or FAISS to find the closest vectors efficiently.
-
Distance Metrics: They support various similarity measures like cosine similarity, Euclidean distance, or dot product.
-
Hybrid Search: Many combine vector similarity with traditional filtering for more precise results.
-
Scalability Features: They're designed to handle billions of vectors while maintaining sub-second query times.
Leading solutions like Pinecone, Weaviate, Milvus, Qdrant, and Chroma each offer different tradeoffs in terms of performance, features, and deployment options.
# Example of storing and querying vectors with Pinecone
import pinecone
# Initialize connection
pinecone.init(api_key="your-api-key", environment="your-environment")
# Create index if it doesn't exist
if "product-embeddings" not in pinecone.list_indexes():
pinecone.create_index("product-embeddings", dimension=1536)
# Connect to the index
index = pinecone.Index("product-embeddings")
# Upsert vectors
index.upsert([
("item1", embedding, {"category": "electronics"}),
# More items...
])
# Query for similar items
results = index.query(
vector=query_embedding,
top_k=5,
filter={"category": "electronics"}
)
Real-World Applications Transformed by Vector Search
Vector databases aren't just academic curiosities—they're powering some of the most impressive AI applications today:
Semantic Search: Moving beyond keyword matching to understanding user intent. Shopify's search now understands that "summer outfit" should return sundresses and shorts, even if those exact words aren't in the product descriptions.
RAG (Retrieval-Augmented Generation): Giving LLMs access to private data without fine-tuning. Companies like Notion and Perplexity use vector search to retrieve relevant documents before generating responses.
Recommendation Engines: Netflix, Spotify, and Amazon all use embedding-based similarity to suggest content or products you might enjoy.
Anomaly Detection: Financial institutions detect unusual patterns by comparing transaction embeddings against known patterns.
Image and Audio Search: Finding visually similar products or music with similar vibes, even when metadata is limited.
Performance Considerations for Production Systems
As you move from prototypes to production, several factors become critical:
Indexing Speed: How quickly can you add new vectors? This matters for real-time applications.
Query Latency: Sub-100ms responses are often necessary for interactive applications.
Recall Accuracy: The percentage of true nearest neighbors returned by approximate algorithms.
Scaling Costs: Vector operations are compute-intensive, making cost optimization important.
For high-performance applications, consider these optimization strategies:
-
Vector Compression: Techniques like Product Quantization can reduce storage requirements by 4-16x with minimal accuracy loss.
-
Filtering Before Vectorization: When possible, use metadata filters to reduce the search space before computing expensive vector similarities.
-
Batching Operations: Group vector operations to amortize overhead costs.
# Optimized batch querying with pre-filtering
results = index.query(
vector=query_embedding,
top_k=100,
filter={"price": {"$lte": 50}},
namespace="in_stock_items"
)
Building Your First Vector-Powered Application
If you're new to vector databases, here's a straightforward path to get started:
-
Choose the Right Embeddings: For text, OpenAI's embeddings offer excellent performance. For images, CLIP or ResNet embeddings work well.
-
Start Small: Begin with a managed service like Pinecone or Qdrant Cloud to avoid operational complexity.
-
Design Your Schema: Consider what metadata you'll need for filtering and how you'll structure your vector spaces.
-
Build a Proof of Concept: Start with a simple semantic search application to understand the workflow.
# A minimal semantic search implementation
from sentence_transformers import SentenceTransformer
import numpy as np
# Load model
model = SentenceTransformer('all-MiniLM-L6-v2')
# Create a small in-memory vector database
documents = [
"Vector databases store embeddings for efficient similarity search",
"Traditional databases use indexes for exact matching",
"Machine learning models convert data into vector representations"
]
# Create embeddings
embeddings = model.encode(documents)
# Simple vector search function
def search(query, top_k=1):
query_embedding = model.encode([query])
# Calculate cosine similarity
similarities = np.dot(embeddings, query_embedding.T).flatten()
# Get top results
indices = similarities.argsort()[-top_k:][::-1]
return [(documents[i], float(similarities[i])) for i in indices]
# Test it
results = search("How do I find similar items?")
print(results)
Conclusion
Vector databases represent a fundamental shift in how we build AI applications, enabling systems that understand similarity and context rather than just exact matches. As embeddings become the universal interface between different AI models and modalities, the importance of efficient vector storage and retrieval will only grow.
Whether you're building a RAG application, a semantic search engine, or a recommendation system, understanding vector databases is no longer optional—it's an essential skill for the modern AI developer. Start small, experiment with different approaches, and you'll quickly discover how these powerful tools can transform your applications from simple pattern-matching to truly intelligent systems that understand the relationships between concepts.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.