Computational Graphs: The Hidden Language Bridging Modern AI and Software Engineering
2025-04-07
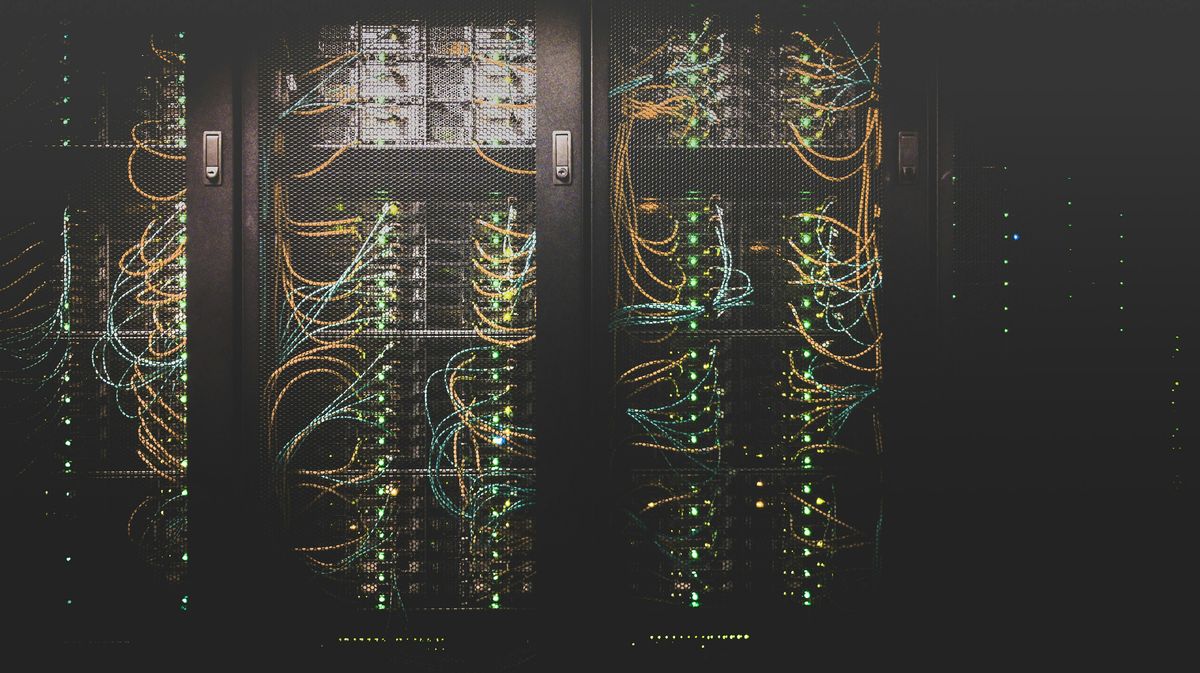
When we talk about AI and traditional software engineering, we often focus on their differences—neural networks versus object-oriented programming, PyTorch versus Java, differentiable versus discrete. But beneath these apparent contrasts lies a unifying abstraction that has quietly become the lingua franca of modern computing: the computational graph. This powerful representation is reshaping how we think about code execution, optimization, and the boundaries between AI and conventional programming.
The Universal Abstraction
At its core, a computational graph is remarkably simple: a directed graph where nodes represent operations and edges represent data flowing between them. This abstraction has become ubiquitous in AI frameworks like TensorFlow and PyTorch, where it enables automatic differentiation and hardware acceleration. But the same concept has been steadily infiltrating traditional software engineering through reactive programming, build systems, and modern compiler designs.
Consider this simple TensorFlow example:
import tensorflow as tf
# Define the computational graph
x = tf.constant(3.0)
y = tf.constant(4.0)
z = x * y + tf.math.sin(x)
# Execute the graph
print(z.numpy()) # Output: 12.141120
The power lies not in the operations themselves, but in how the framework can analyze, transform, and optimize the entire structure before execution. This same principle is now appearing in build systems like Bazel, stream processing frameworks like Apache Beam, and even JavaScript's reactive libraries.
From AI to Software Engineering: The Cross-Pollination
The computational graph abstraction didn't stay confined to AI. Modern software engineering has been increasingly adopting graph-based execution models for entirely different reasons:
-
Reactive Programming: Libraries like RxJS and frameworks like React represent computation as data flow graphs, enabling elegant handling of asynchronous events.
-
Build Systems: Tools like Bazel and Buck model build dependencies as graphs, allowing for intelligent incremental compilation and parallel execution.
-
Data Processing: Apache Spark, Flink, and Beam all represent data transformations as computational graphs that can be optimized and distributed.
This JavaScript example using RxJS demonstrates how mainstream programming has embraced graph-based thinking:
import { fromEvent, merge } from 'rxjs';
import { map, debounceTime } from 'rxjs/operators';
// Create a computational graph for UI events
const mouseMove$ = fromEvent(document, 'mousemove').pipe(
map(event => ({ x: event.clientX, y: event.clientY })),
debounceTime(50)
);
const keyPress$ = fromEvent(document, 'keypress').pipe(
map(event => event.key)
);
// Combine streams in the graph
merge(mouseMove$, keyPress$).subscribe(data => {
console.log('User interaction:', data);
});
The Compiler Revolution
Perhaps the most profound impact of computational graphs is happening in compiler technology. Traditional compilers like LLVM have long used graph-based intermediate representations, but now they're being reimagined to handle both AI and traditional code through unified abstractions.
MLIR (Multi-Level Intermediate Representation) exemplifies this trend, allowing different programming models to share optimization techniques. Similarly, Julia's compiler uses a sophisticated computational graph to perform type inference and optimization across both numerical and general-purpose code.
# Julia's compiler builds computational graphs behind the scenes
function matrix_operations(A, x)
# These operations form a computational graph that Julia optimizes
b = A * x
c = sum(b.^2)
return sqrt(c)
end
# Works efficiently with both standard arrays and AI tensor libraries
result = matrix_operations(rand(1000, 1000), rand(1000))
Performance Convergence
The computational graph abstraction has led to remarkable performance convergence between AI and traditional software. Techniques originally developed for one domain are crossing over:
-
Just-in-Time Compilation: Techniques pioneered in JavaScript engines are now used in PyTorch's eager execution mode.
-
Parallelization: Graph-based automatic parallelization strategies from TensorFlow are inspiring new approaches in general-purpose compilers.
-
Memory Management: Sophisticated buffer reuse algorithms from deep learning frameworks are influencing memory allocators in systems programming.
This cross-pollination is creating a virtuous cycle of optimization, where advances in one field benefit the other.
Developer Experience Transformation
Beyond performance, computational graphs are transforming the developer experience. By making data flow explicit, they enable powerful new tools:
-
Visualization: Tools like TensorBoard allow developers to visualize and debug complex execution flows.
-
Profiling: Graph-based profilers can identify bottlenecks at a higher level of abstraction.
-
Automated Optimization: Compilers can perform sophisticated transformations that would be impossible with traditional code.
This shift is particularly valuable as systems grow more complex and distributed, providing a mental model that scales better than imperative programming alone.
The Future: Graph-Native Development
As computational graphs become more prevalent, we're seeing the emergence of "graph-native" development approaches that blur the line between AI and traditional programming:
-
Differentiable Programming: Languages like Swift for TensorFlow and Jax are making differentiation a first-class operation in general-purpose languages.
-
Graph DSLs: Domain-specific languages built around computational graphs, like Apache Beam's pipeline DSL, are providing high-level abstractions with sophisticated optimizations.
-
Hybrid Execution Models: Systems that seamlessly mix traditional and AI workloads, optimizing across the boundary.
Conclusion
The computational graph has emerged as a powerful unifying abstraction that transcends the traditional boundaries between AI and software engineering. As these worlds continue to converge, developers who understand graph-based thinking will have an advantage in building the next generation of intelligent systems.
Rather than viewing AI and traditional programming as separate disciplines, we're entering an era where computational graphs provide a common language for expressing computation—regardless of whether it's training a neural network or processing a stream of user events. This convergence promises not just better performance and more sophisticated tools, but a fundamentally more powerful way to think about software development in a world where intelligence is becoming embedded in every application.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.