Differentiable Programming: When AI Learns to Write Its Own Algorithms
2025-04-13
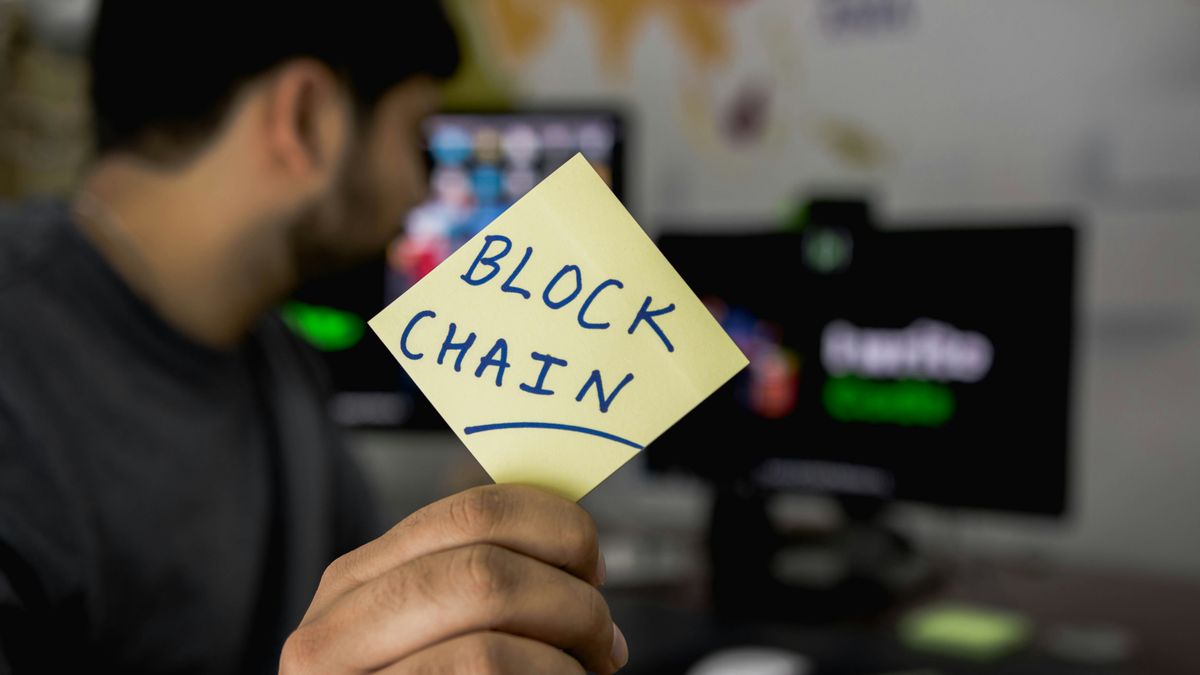
For decades, we've maintained a clear separation: humans write algorithms, machines execute them. But what if the machines could refine—or even create—those algorithms themselves? Differentiable programming is dissolving this boundary, allowing us to build software that can optimize itself through learning. It's not just a new programming paradigm; it's potentially the future of how we'll develop intelligent systems in an increasingly complex computational world.
The Gradient Revolution
At its core, differentiable programming is about making every component of a program "differentiable"—capable of computing gradients that show how small changes in inputs affect outputs. This seemingly simple mathematical property unlocks extraordinary power: the ability for programs to optimize themselves through gradient descent, the same principle that drives deep learning.
Traditional programming is explicit and deterministic: we specify exactly what should happen for every input. Differentiable programming, however, creates systems that can tune themselves based on data and desired outcomes. Consider this simple example:
# Traditional approach: Explicitly coded function
def traditional_scale(data, factor=2.0):
return data * factor
# Differentiable approach: Learnable parameter
import torch
class DifferentiableScaler(torch.nn.Module):
def __init__(self):
super().__init__()
# Parameter that can be optimized through gradient descent
self.factor = torch.nn.Parameter(torch.tensor(2.0))
def forward(self, data):
return data * self.factor
# The factor can now be optimized based on data
The difference might seem subtle, but it's profound. In the second example, the scaling factor isn't fixed—it can be optimized automatically based on the data and desired outcomes.
Beyond Deep Learning
While neural networks are inherently differentiable, differentiable programming extends this property to traditional algorithms and data structures. Libraries like JAX, PyTorch, and TensorFlow now allow us to make almost any computation differentiable.
Consider sorting, a fundamental algorithm in computer science. Traditional sorting is non-differentiable due to its discrete nature. However, we can create differentiable approximations:
# Using a differentiable sorting approximation in JAX
import jax
import jax.numpy as jnp
def soft_sort(x, temperature=0.1):
"""A differentiable approximation of sorting."""
x_sorted = jnp.sort(x)
# Create pairwise distances for all elements
x_ext = jnp.expand_dims(x, 1)
diffs = jnp.abs(x_ext - jnp.expand_dims(x_sorted, 0))
# Convert distances to probabilities
weights = jax.nn.softmax(-diffs / temperature, axis=1)
# Weighted sum gives differentiable approximation
return jnp.sum(weights * jnp.expand_dims(x_sorted, 0), axis=1)
This "soft" version of sorting produces gradients that allow the inputs to be optimized. Similar techniques have been applied to other traditionally non-differentiable operations like argmax, if-statements, and even graph algorithms.
Hybrid Systems: The Best of Both Worlds
Perhaps the most exciting applications come from combining traditional algorithms with differentiable components. These hybrid systems leverage human expertise in algorithm design while allowing machine learning to tune specific parameters or components.
Take pathfinding algorithms. We might encode our knowledge of A* search but make the heuristic function differentiable:
import torch
class LearnableHeuristic(torch.nn.Module):
def __init__(self, feature_dim):
super().__init__()
self.network = torch.nn.Sequential(
torch.nn.Linear(feature_dim, 64),
torch.nn.ReLU(),
torch.nn.Linear(64, 1)
)
def forward(self, state_features):
return self.network(state_features)
# The A* algorithm remains the same, but now uses
# a neural network to estimate distances to goal
By training on successful paths, the heuristic function improves over time, making the algorithm more efficient without sacrificing its guarantees.
Automatic Algorithm Design
One of the most fascinating applications is using differentiable programming to discover algorithms automatically. Rather than hand-crafting specialized algorithms, we can define a differentiable template and let gradient descent find the optimal configuration.
For instance, researchers have used this approach to discover image processing algorithms:
import torch
class LearnableImageFilter(torch.nn.Module):
def __init__(self):
super().__init__()
# Learnable convolutional kernels
self.kernels = torch.nn.Parameter(torch.randn(5, 3, 3, 3))
self.weights = torch.nn.Parameter(torch.ones(5) / 5)
def forward(self, image):
results = []
for k in range(5):
# Apply each kernel
filtered = torch.nn.functional.conv2d(
image, self.kernels[k:k+1], padding=1
)
results.append(filtered)
# Weighted combination of filter outputs
return sum(w * r for w, r in zip(self.weights, results))
When trained on appropriate data, such systems can discover specialized algorithms that outperform hand-crafted solutions for specific tasks. In some cases, they've even rediscovered classic algorithms like Sobel edge detection—but optimized for particular applications.
The Future: Self-Improving Software
As differentiable programming matures, we're moving toward a future where software doesn't just execute—it evolves. Imagine deployment pipelines where applications continuously improve based on real-world usage data, automatically optimizing their algorithms without explicit reprogramming.
This doesn't mean programmers become obsolete. Rather, our role shifts from writing explicit algorithms to designing differentiable architectures that can learn and adapt. The programmer becomes more of an architect, creating spaces of possible programs rather than single, fixed implementations.
Consider this evolution in how we might approach a recommendation system:
# Traditional approach
def recommend(user_id, item_catalog):
user_preferences = get_user_data(user_id)
scores = []
for item in item_catalog:
# Hard-coded scoring function
score = compute_similarity(user_preferences, item)
scores.append((item, score))
return sorted(scores, key=lambda x: x[1], reverse=True)[:10]
# Differentiable approach
class DifferentiableRecommender(torch.nn.Module):
def __init__(self):
super().__init__()
# Architecture that can learn from user interactions
self.user_encoder = torch.nn.Sequential(...)
self.item_encoder = torch.nn.Sequential(...)
self.interaction = torch.nn.Bilinear(...)
def forward(self, user_features, item_features):
user_emb = self.user_encoder(user_features)
item_emb = self.item_encoder(item_features)
return self.interaction(user_emb, item_emb)
The differentiable version doesn't just execute a fixed algorithm—it learns what makes recommendations effective based on user feedback.
Conclusion
Differentiable programming represents a fundamental shift in how we approach software development. By making computation itself learnable, we're creating systems that can adapt and improve automatically. The line between "programming" and "training" is blurring, giving rise to a new paradigm where code isn't just written—it's evolved.
As we continue to develop this approach, we'll likely see increasingly sophisticated hybrid systems that combine human-designed algorithmic structures with machine-learned components. The future of programming may not be writing instructions for computers, but rather designing differentiable architectures that can discover their own optimal algorithms.
For developers, this means expanding our toolkit beyond traditional software engineering to include gradient-based optimization and machine learning. The most powerful systems of tomorrow will be those that can leverage both human expertise and the optimization capabilities of differentiable programming—creating software that improves itself.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.