Differentiable Programming: When Code Learns to Optimize Itself
2025-04-09
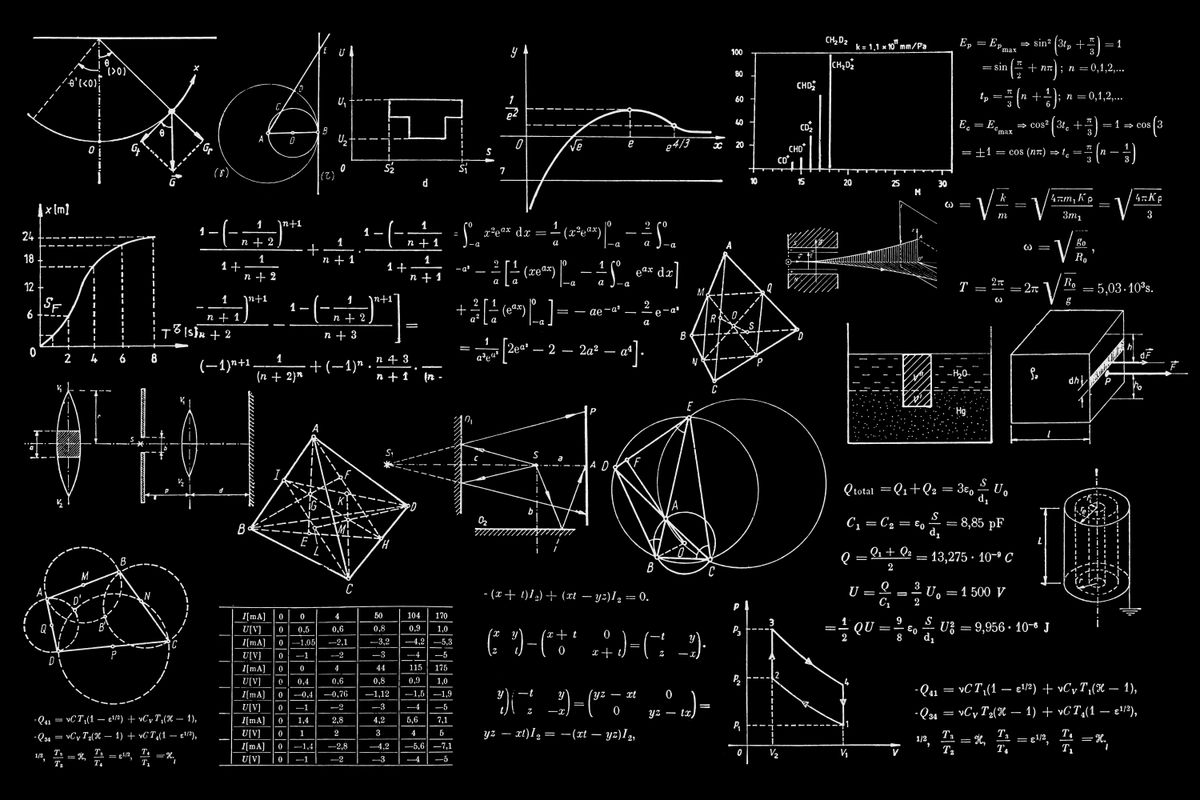
The boundaries between traditional programming and artificial intelligence are dissolving. At this fascinating intersection stands differentiable programming—a paradigm that's revolutionizing how we think about software development. Unlike conventional programming where logic is explicitly defined, differentiable programming creates systems that can learn from data, adapt to new scenarios, and optimize themselves through gradient-based methods. This approach isn't just reshaping AI; it's fundamentally changing how we conceptualize software engineering itself.
The Evolution from Fixed Logic to Learnable Programs
Traditional software engineering relies on developers explicitly coding every rule and condition. We write precise instructions for computers to follow:
def calculate_shipping(weight, distance):
base_rate = 5.00
weight_factor = 0.75 * weight
distance_factor = 0.25 * distance
return base_rate + weight_factor + distance_factor
This approach works well for problems with clear rules, but struggles with complexity and adaptation. Differentiable programming takes a different approach by creating programs where some components can be automatically optimized:
def calculate_shipping(weight, distance, params):
base_rate = params[0]
weight_factor = params[1] * weight
distance_factor = params[2] * distance
return base_rate + weight_factor + distance_factor
# The parameters can be learned from shipping data
# using gradient descent to minimize shipping cost errors
By making our functions differentiable, we can use gradient-based optimization to find the optimal parameters, creating code that adapts to real-world data rather than relying solely on human intuition.
The Mathematics Behind Learnable Code
At its core, differentiable programming relies on calculating derivatives of program outputs with respect to their parameters. This seemingly simple mathematical concept enables software to learn from experience.
Consider a neural network layer, which is fundamentally a differentiable function:
def linear_layer(x, weights, bias):
return np.dot(x, weights) + bias
The magic happens when we chain these differentiable functions together and use automatic differentiation to compute gradients throughout the entire program. Modern frameworks like JAX, PyTorch, and TensorFlow have made this process remarkably accessible:
import jax
import jax.numpy as jnp
def model(params, x):
# A simple two-layer neural network
w1, b1, w2, b2 = params
hidden = jnp.tanh(jnp.dot(x, w1) + b1)
output = jnp.dot(hidden, w2) + b2
return output
# Compute gradients automatically
gradient_function = jax.grad(model, argnums=0)
This ability to automatically compute gradients through arbitrary code transforms how we approach optimization problems across domains.
Beyond Neural Networks: Differentiable Algorithms
While neural networks are the most recognizable form of differentiable programming, the paradigm extends far beyond them. Researchers are now creating differentiable versions of classical algorithms, from sorting and searching to graph algorithms and physics simulations.
Consider a differentiable sorting operation. Traditional sorting is non-differentiable because small changes in inputs can cause discrete jumps in the output order. However, by creating continuous relaxations of sorting, we can approximate it in a differentiable manner:
def soft_sort(x, temperature=1.0):
"""A differentiable approximation of sorting"""
x_sorted = jnp.sort(x)
weights = jax.nn.softmax(-jnp.abs(x - x_sorted[:, None]) / temperature)
return jnp.sum(weights * x_sorted[:, None], axis=0)
This approach allows us to incorporate sorting operations within end-to-end differentiable pipelines, enabling optimization of systems that include sorting as a component.
Practical Applications in Modern Software
Differentiable programming is already transforming real-world applications across industries:
-
Computer Graphics: Differentiable renderers enable optimization of 3D models by backpropagating through the rendering process.
-
Robotics: Robot control policies can be learned by differentiating through physics simulations.
-
Database Systems: Query optimizers can learn from execution statistics by making the query planning process differentiable.
-
Compiler Optimization: Compilers can learn to generate more efficient code by differentiating through performance metrics.
For example, a differentiable physics engine allows us to optimize robot designs:
def simulate_robot(design_params, control_inputs):
# Create robot model from design parameters
robot = create_robot_model(design_params)
# Simulate robot movement with physics
final_state = differentiable_physics_simulation(robot, control_inputs)
# Return some performance metric
return compute_performance(final_state)
# Optimize robot design
optimal_design = gradient_descent(simulate_robot, initial_design)
This enables a new design paradigm where physical systems can be optimized end-to-end for specific tasks.
The Future: Hybrid Programming Models
As differentiable programming matures, we're moving toward hybrid systems that combine traditional software engineering with learnable components. This fusion leverages the strengths of both approaches: the reliability and interpretability of explicit logic with the adaptability and optimization capabilities of differentiable systems.
Future programming languages might include gradient-based optimization as a first-class feature:
# Hypothetical future programming language
@differentiable
class ShippingCalculator:
def __init__(self):
# Parameters that can be optimized
self.base_rate = Parameter(5.0)
self.weight_coefficient = Parameter(0.75)
self.distance_coefficient = Parameter(0.25)
def calculate(self, weight, distance):
return (self.base_rate +
self.weight_coefficient * weight +
self.distance_coefficient * distance)
# Optimize parameters using historical shipping data
calculator = ShippingCalculator()
calculator.optimize(training_data, loss_function='mean_squared_error')
This integration will make machine learning capabilities accessible to mainstream developers without requiring deep expertise in AI.
Conclusion
Differentiable programming represents a fundamental shift in how we create software. By making programs learnable through gradient-based optimization, we're building systems that can adapt and improve based on real-world data. This paradigm doesn't replace traditional programming—it extends it, offering new tools for tackling problems that were previously intractable.
As this field evolves, we can expect to see more software components becoming differentiable, more powerful optimization techniques, and more intuitive tools that make these capabilities accessible to all developers. The line between "writing code" and "training models" will continue to blur, creating a new generation of adaptive, self-optimizing software systems that combine the best of human ingenuity and machine learning.
The future of programming isn't just about telling computers what to do—it's about creating systems that can learn how to do things better.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.