Federated Learning: Privacy-Preserving AI in a Data-Hungry World
2025-04-11
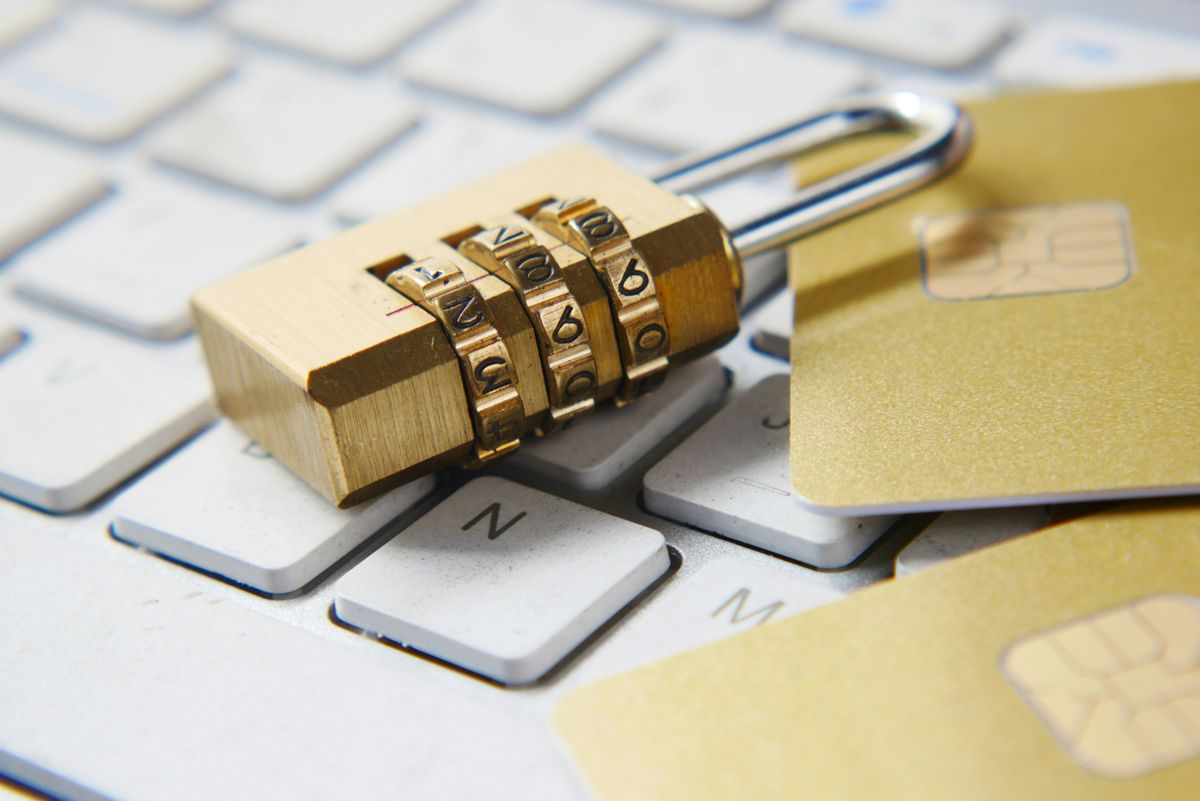
In our increasingly connected digital ecosystem, the tension between data privacy and AI advancement has reached a critical point. Traditional machine learning approaches demand centralized data collection—often raising serious privacy concerns and regulatory challenges. Enter federated learning: a paradigm-shifting approach that allows models to learn from distributed datasets without ever moving sensitive information from its source. This revolutionary technique doesn't just solve a technical problem; it fundamentally reframes the relationship between AI systems and the data they learn from.
The Core Mechanics of Federated Learning
At its heart, federated learning inverts the traditional ML workflow. Rather than bringing data to the model, it brings the model to the data. The process follows a surprisingly elegant pattern:
- A central server distributes the current model to participating devices
- Each device trains the model on its local data
- Only model updates (not raw data) are sent back to the server
- The server aggregates these updates to improve the global model
This approach creates a collaborative learning environment while maintaining data sovereignty. Here's a simplified implementation using TensorFlow Federated:
import tensorflow as tf
import tensorflow_federated as tff
# Define a simple model function
def create_model():
return tf.keras.models.Sequential([
tf.keras.layers.Dense(10, activation=tf.nn.relu, input_shape=(784,)),
tf.keras.layers.Dense(10, activation=tf.nn.softmax)
])
# Define client training function
def client_update(model, dataset, epochs):
model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.SGD(0.1),
metrics=['accuracy'])
model.fit(dataset, epochs=epochs)
return model.weights
# Federated averaging algorithm (simplified)
def federated_avg(weights_list):
# Average weights from all clients
return tf.nest.map_structure(
lambda *weights: tf.reduce_mean(tf.stack(weights, axis=0), axis=0),
*weights_list)
This conceptual implementation demonstrates how a model can learn collaboratively while keeping raw data private—a fundamental shift from traditional approaches.
Real-World Applications Transforming Industries
Federated learning isn't just theoretical—it's already transforming how organizations approach sensitive data challenges:
Healthcare: Medical institutions can collaboratively train diagnostic models across hospitals without sharing patient records. A consortium of cancer centers might develop more accurate tumor detection algorithms while maintaining strict HIPAA compliance.
Mobile Keyboards: Google's Gboard uses federated learning to improve next-word prediction without sending your typing data to the cloud. The keyboard learns your personal writing style while keeping sensitive text on your device.
Financial Services: Banks can build fraud detection systems that learn from transaction patterns across institutions without exposing customer financial data.
IoT Networks: Smart home devices can collectively improve their intelligence without sending potentially sensitive behavioral data to the cloud.
The pattern is clear: federated learning thrives where data privacy concerns would otherwise limit AI advancement.
Technical Challenges and Innovative Solutions
Despite its promise, federated learning introduces unique technical hurdles that researchers are actively addressing:
Communication Efficiency: Sending model updates across networks can create significant bandwidth overhead. Techniques like model compression and update quantization have emerged as solutions:
# Example of gradient quantization for efficient communication
def quantize_gradients(gradients, bits=8):
# Find the maximum absolute value
max_abs = tf.reduce_max(tf.abs(gradients))
# Scale to the range [-2^(bits-1), 2^(bits-1)-1]
scale = (2**(bits-1) - 1) / max_abs
quantized = tf.cast(tf.round(gradients * scale), tf.int8)
# Return quantized gradients and scale for dequantization
return quantized, max_abs
Statistical Heterogeneity: Client data is often non-IID (not independently and identically distributed), creating training challenges. Techniques like FedProx add regularization terms to mitigate this problem:
# FedProx implementation with proximal term
def fedprox_client_update(model, dataset, global_weights, mu=0.01):
# Standard training
with tf.GradientTape() as tape:
predictions = model(data)
loss = loss_fn(labels, predictions)
# Add proximal term to penalize deviation from global model
proximal_term = 0
for w, w_global in zip(model.weights, global_weights):
proximal_term += tf.reduce_sum(tf.square(w - w_global))
# Add regularized proximal term to loss
total_loss = loss + (mu/2) * proximal_term
# Compute gradients and update model
gradients = tape.gradient(total_loss, model.trainable_variables)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
Security Concerns: Even without sharing raw data, model updates can potentially leak information. Differential privacy techniques add carefully calibrated noise to protect against inference attacks:
# Simplified differential privacy implementation
def apply_differential_privacy(gradients, noise_multiplier=1.0, l2_norm_clip=1.0):
# Clip gradients to bound sensitivity
gradients_flat = tf.concat([tf.reshape(g, [-1]) for g in gradients], axis=0)
l2_norm = tf.norm(gradients_flat)
scale = tf.minimum(1.0, l2_norm_clip / l2_norm)
gradients = [g * scale for g in gradients]
# Add calibrated Gaussian noise
noise_stddev = l2_norm_clip * noise_multiplier
noisy_gradients = [g + tf.random.normal(shape=tf.shape(g),
stddev=noise_stddev)
for g in gradients]
return noisy_gradients
These innovations are steadily removing barriers to federated learning adoption, expanding its practical applications.
The Privacy-Utility Frontier
Federated learning represents a fundamental rethinking of the privacy-utility tradeoff in machine learning. Rather than viewing privacy as a constraint that limits model capability, it positions privacy as a design principle that can coexist with powerful AI.
This shift has profound implications for how we conceptualize data ownership. In traditional ML, organizations effectively claim ownership of user data through centralized collection. Federated learning, by contrast, respects data sovereignty—allowing individuals and organizations to contribute to model improvement without surrendering control of their information.
This approach aligns perfectly with emerging regulatory frameworks like GDPR and CCPA, which emphasize data minimization and purpose limitation. By processing data where it originates, federated learning satisfies both the letter and spirit of modern privacy laws.
Building a Federated Future
As federated learning matures, we're seeing the emergence of robust frameworks and tools that democratize access to these techniques:
TensorFlow Federated (TFF): Google's open-source framework provides high-level APIs for experimenting with federated learning algorithms.
PySyft: A library that extends PyTorch with privacy-preserving capabilities, including federated learning.
FATE (Federated AI Technology Enabler): An industrial-grade federated learning framework with a focus on secure computation.
To start experimenting with federated learning today, consider this minimal TFF example:
import tensorflow as tf
import tensorflow_federated as tff
# Load and preprocess the MNIST dataset
def preprocess_dataset():
mnist = tf.keras.datasets.mnist
(x_train, y_train), _ = mnist.load_data()
x_train = x_train.reshape(60000, 784).astype('float32') / 255
return x_train, y_train
# Create TFF datasets from MNIST
def create_federated_datasets(x, y, num_clients=10):
# Simulate data distribution across clients
samples_per_client = len(x) // num_clients
client_datasets = []
for i in range(num_clients):
start = i * samples_per_client
end = (i + 1) * samples_per_client
client_data = tf.data.Dataset.from_tensor_slices(
(x[start:end], y[start:end])).batch(20)
client_datasets.append(client_data)
return client_datasets
# Initialize the federated learning process
x, y = preprocess_dataset()
federated_train_data = create_federated_datasets(x, y)
# Define model and federated averaging process
# (TFF handles the federated computation details)
Conclusion
Federated learning represents one of the most significant paradigm shifts in machine learning—not just as a technical innovation, but as a philosophical reorientation that places privacy at the center of AI development. By enabling models to learn from distributed data without centralized collection, it creates a path forward for AI advancement in domains where privacy concerns have previously created insurmountable barriers.
As regulations tighten and consumers become increasingly privacy-conscious, federated learning isn't just a nice-to-have—it's becoming essential infrastructure for responsible AI development. The organizations that embrace this approach now will be well-positioned to build powerful, privacy-preserving systems that earn user trust while delivering exceptional performance.
The future of AI isn't about amassing the largest possible datasets in centralized repositories. It's about building intelligent systems that respect boundaries, preserve privacy, and still deliver remarkable capabilities. Federated learning is leading this transformation, one distributed update at a time.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.