Probabilistic Programming: When Uncertainty Becomes a Feature, Not a Bug
2025-04-10
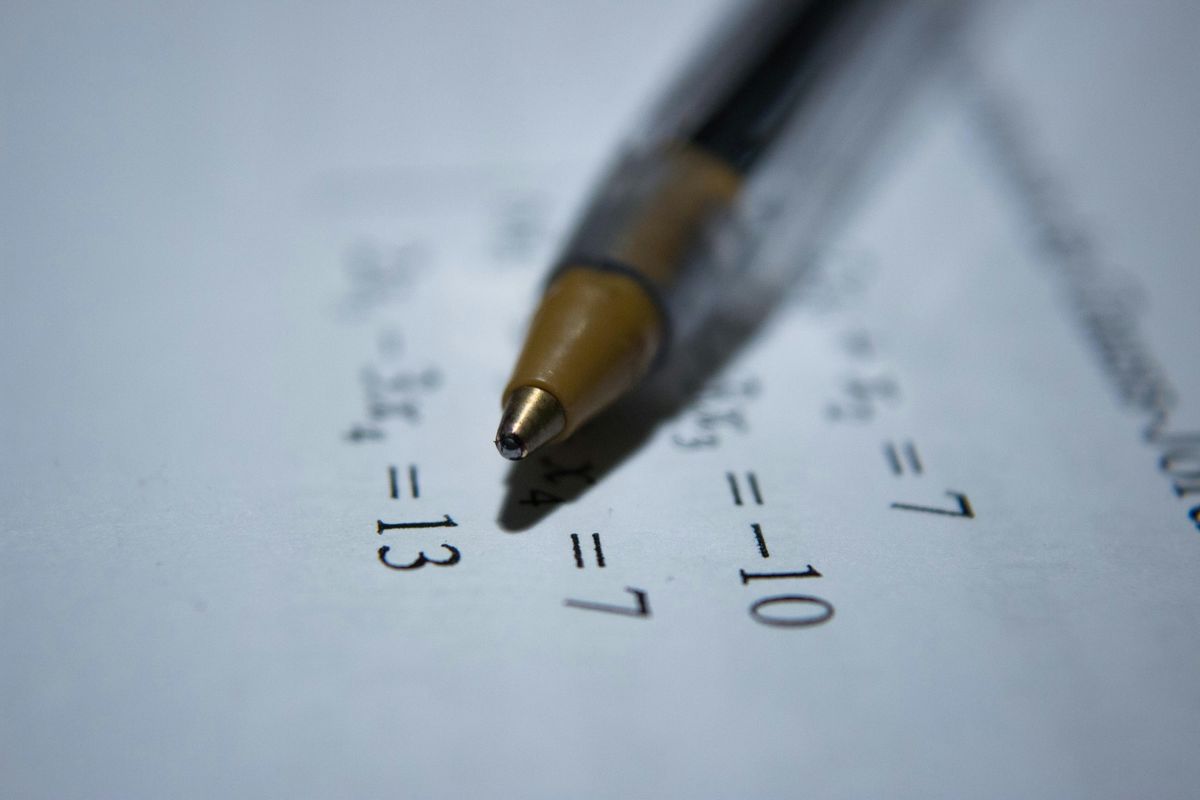
In traditional software development, uncertainty has always been the enemy. We build systems with precise logic, deterministic outcomes, and explicit error handling. But what if uncertainty isn't a limitation to overcome, but a powerful feature to embrace? This is the paradigm shift offered by probabilistic programming—a revolutionary approach that's transforming how we develop AI systems by encoding uncertainty directly into our code.
The Uncertainty Revolution
We live in an uncertain world. Our sensors are noisy, our data is incomplete, and the phenomena we model are inherently stochastic. Traditional programming paradigms force us to abstract away this uncertainty, often resulting in brittle systems that fail when confronted with edge cases or ambiguity.
Probabilistic programming languages (PPLs) flip this script by making uncertainty a first-class citizen in code. Rather than writing programs that produce single, deterministic outputs, PPLs enable us to write models that express distributions over possible outcomes.
# Traditional programming: deterministic
def predict_weather(temperature, pressure, humidity):
if temperature > 30 and humidity > 0.8:
return "Rain"
else:
return "No rain"
# Probabilistic programming: handles uncertainty
def predict_weather_prob(temperature, pressure, humidity):
# Parameters have uncertainty
temp = Normal(temperature, 1.0) # Sensor has ±1° error
hum = Normal(humidity, 0.05) # Humidity sensor has 5% error
# Model returns a probability distribution
rain_prob = sigmoid(3.0 * temp + 2.0 * hum - 95.0)
return Bernoulli(rain_prob) # Distribution over "Rain" or "No rain"
Bridging Statistical Modeling and Software Engineering
At its core, probabilistic programming represents a convergence of two disciplines that historically operated in separate domains: statistical modeling and software engineering.
Statisticians and data scientists have long built models that capture uncertainty, but implementing these models often required specialized knowledge and custom code. Software engineers, meanwhile, excel at building modular, maintainable systems, but traditional programming languages lack native constructs for handling probabilistic reasoning.
PPLs bridge this gap by providing the expressiveness of programming languages with the statistical rigor of Bayesian modeling frameworks. This fusion enables a new class of applications that can reason about uncertainty while leveraging software engineering best practices.
# PyMC3 example: Bayesian linear regression
import pymc3 as pm
import numpy as np
# Generate synthetic data
X = np.random.normal(0, 1, size=100)
y = 2 * X + np.random.normal(0, 0.5, size=100)
with pm.Model() as linear_model:
# Prior distributions for parameters
alpha = pm.Normal('alpha', mu=0, sigma=10)
beta = pm.Normal('beta', mu=0, sigma=10)
sigma = pm.HalfNormal('sigma', sigma=1)
# Expected value of outcome
mu = alpha + beta * X
# Likelihood (sampling distribution) of observations
y_obs = pm.Normal('y_obs', mu=mu, sigma=sigma, observed=y)
# Inference
trace = pm.sample(1000, tune=1000)
# We now have distributions over alpha, beta, and sigma
The Inference Engine: Where the Magic Happens
The true power of probabilistic programming lies in automatic inference. When we write a probabilistic program, we're defining a generative model—a process that describes how observed data might have been generated. The inference engine then works backward from observations to infer the distribution of unobserved variables.
This automatic inference is what makes PPLs so revolutionary. Instead of hand-coding algorithms to estimate parameters, the PPL handles this complexity, allowing developers to focus on model specification rather than inference mechanics.
Different PPLs employ various inference strategies:
# TensorFlow Probability: Variational inference
import tensorflow as tf
import tensorflow_probability as tfp
tfd = tfp.distributions
def variational_model():
return tfd.JointDistributionSequential([
tfd.Normal(loc=0., scale=1., name="weight"),
tfd.Normal(loc=0., scale=1., name="bias"),
lambda bias, weight: tfd.Normal(
loc=weight * x + bias, scale=1., name="observation")
])
# Define variational posterior
def variational_posterior():
return tfd.JointDistributionSequential([
tfd.Normal(loc=tf.Variable(0.), scale=tf.Variable(1.)),
tfd.Normal(loc=tf.Variable(0.), scale=tf.Variable(1.)),
])
# Run variational inference
losses = tfp.vi.fit_surrogate_posterior(
variational_posterior,
variational_model,
num_steps=1000
)
Real-World Applications: From Recommendation to Robotics
Probabilistic programming is transforming AI applications across domains. Let's explore a few compelling use cases:
Recommendation Systems
Traditional recommendation systems often struggle with the "cold start" problem and uncertainty in user preferences. Probabilistic approaches naturally handle these challenges by modeling user preferences as distributions rather than point estimates.
# Simple Bayesian recommendation model in Edward2
import edward2 as ed
import tensorflow as tf
def recommendation_model(num_users, num_items, latent_dim):
# User embeddings with priors
user_factors = ed.Normal(
loc=tf.zeros([num_users, latent_dim]),
scale=tf.ones([num_users, latent_dim]),
name="user_factors")
# Item embeddings with priors
item_factors = ed.Normal(
loc=tf.zeros([num_items, latent_dim]),
scale=tf.ones([num_items, latent_dim]),
name="item_factors")
# Ratings as dot products with noise
def rating_likelihood(user_id, item_id):
u_factors = tf.gather(user_factors, user_id)
i_factors = tf.gather(item_factors, item_id)
return ed.Normal(
loc=tf.reduce_sum(u_factors * i_factors, axis=1),
scale=0.1,
name="rating")
return rating_likelihood
Computer Vision Under Uncertainty
Computer vision systems often need to make decisions with partial information. Probabilistic programming allows these systems to express confidence in their predictions and take appropriate actions based on uncertainty levels.
Robotics and Control
Robots operate in noisy, unpredictable environments. Probabilistic programming enables robots to reason about uncertainty in sensor readings, environmental dynamics, and the effects of their actions.
The Ecosystem: Tools of the Trade
The probabilistic programming ecosystem has matured significantly in recent years. Here are some of the leading frameworks:
- PyMC3/PyMC4: Python-based PPLs focused on statistical modeling with automatic differentiation variational inference (ADVI) and MCMC sampling
- Stan: A statically-typed PPL with a C++ backend, known for its efficient Hamiltonian Monte Carlo implementation
- TensorFlow Probability: Google's library integrating probabilistic reasoning with deep learning
- Pyro/NumPyro: Uber's PPL built on PyTorch, specializing in variational inference
- Gen: Julia-based PPL focused on flexibility in inference strategies
Each framework has its strengths, but they all share the common goal of making probabilistic reasoning accessible to developers.
# Gen example: A simple Bayesian network
@gen function simple_model()
cloudy = @trace(bernoulli(0.3), :cloudy)
# Probability of rain depends on whether it's cloudy
rain_prob = cloudy ? 0.8 : 0.2
rain = @trace(bernoulli(rain_prob), :rain)
# Probability of sprinkler depends on whether it's cloudy
sprinkler_prob = cloudy ? 0.1 : 0.4
sprinkler = @trace(bernoulli(sprinkler_prob), :sprinkler)
# Grass will be wet if either sprinkler is on or it's raining
grass_wet_prob = if rain && sprinkler
0.99
elseif rain
0.9
elseif sprinkler
0.8
else
0.1
end
grass_wet = @trace(bernoulli(grass_wet_prob), :grass_wet)
return grass_wet
end
# Perform inference given that grass is wet
observations = choicemap((:grass_wet, true))
(trace, _) = generate(simple_model, (), observations)
Conclusion
Probabilistic programming represents a fundamental shift in how we approach software development for AI systems. By embracing uncertainty rather than fighting it, we can build more robust, adaptable, and realistic models of the world.
As the field matures, we're witnessing the democratization of Bayesian modeling—once the domain of specialists with deep statistical knowledge, now accessible to software engineers through intuitive programming interfaces. This convergence of disciplines is unlocking new possibilities across domains, from healthcare to finance, robotics to recommendation systems.
The future of AI isn't just about deterministic algorithms that produce single answers; it's about systems that reason with uncertainty, express confidence in their predictions, and adapt as new information becomes available. Probabilistic programming is the bridge to that future—a future where uncertainty isn't a bug to be fixed, but a feature to be leveraged.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.