Prompting for Programmers: How to Collaborate Effectively with AI Coding Assistants
2025-04-02
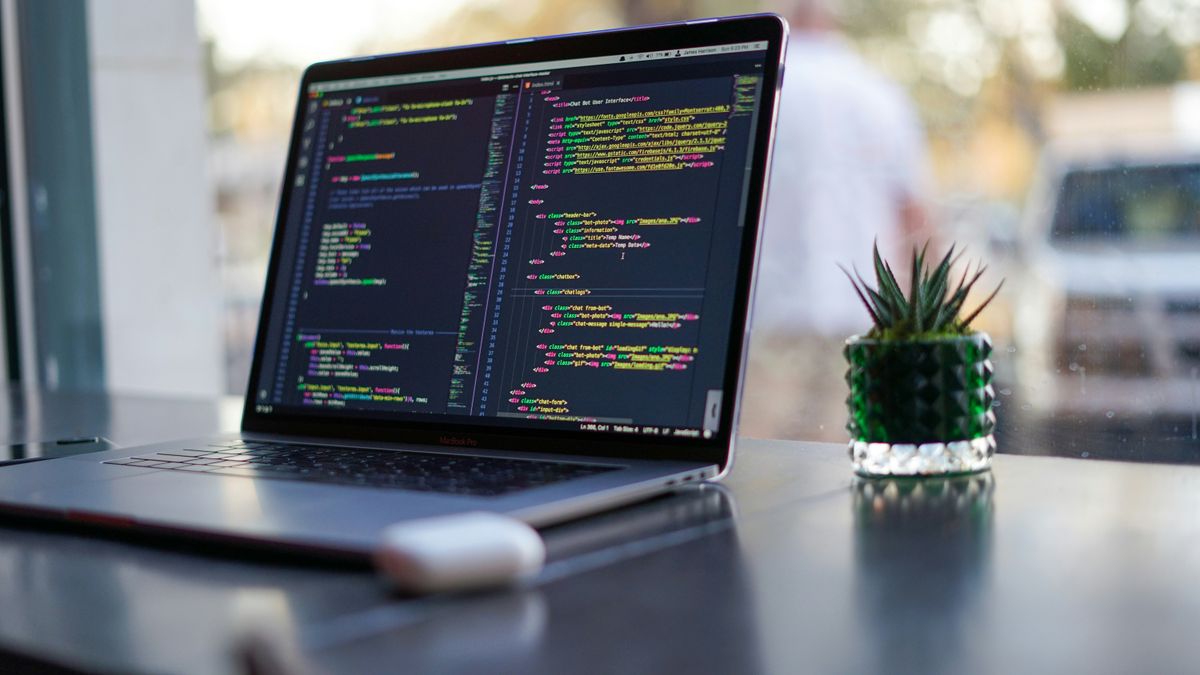
The relationship between developers and AI tools has evolved from novelty to necessity. As coding assistants become more sophisticated, the difference between mediocre and exceptional results often comes down to one thing: how effectively you communicate with your AI partner. Mastering the art of prompting isn't just about getting code snippets faster—it's about unlocking a truly collaborative workflow that amplifies your capabilities as a developer.
Understanding the Prompt-Response Paradigm
AI coding assistants operate on a fundamental input-output model: the quality of what you get out depends heavily on what you put in. Unlike human pair programmers who can infer context from minimal information, AI requires deliberate framing to deliver its best work.
Consider these two approaches to the same problem:
// Ineffective prompt:
"Write a function to sort data"
// Effective prompt:
"Write a TypeScript function that sorts an array of user objects by their 'lastActive' date property, with the most recent first. Each user object has the structure: { id: string, name: string, lastActive: Date }. Include error handling for invalid inputs."
The second prompt provides crucial context: the language (TypeScript), data structure (array of user objects), sorting criteria (lastActive date), direction (most recent first), and additional requirements (error handling). This specificity guides the AI toward generating precisely what you need, saving you from extensive rewrites.
Iterative Refinement: The Conversation Approach
The most powerful interactions with AI coding assistants aren't one-off requests but ongoing conversations. Start with a high-level prompt, evaluate the response, then refine with additional context or corrections.
Here's how an iterative conversation might flow:
// Initial prompt:
"Create a React component that displays a paginated list of items"
// AI generates a basic component
// Follow-up prompt:
"Great start. Now modify it to handle loading states and add error handling when the data fetch fails."
// AI refines the component
// Further refinement:
"Perfect. Can you add TypeScript typing and make the component accept a custom rendering function for each item as a prop?"
This conversational approach mirrors natural human collaboration and leads to progressively better results with each iteration.
Context Is King: Providing Background Information
AI coding assistants lack access to your full development environment. Providing relevant context about your project dramatically improves the relevance of generated code.
Effective context includes:
- Technology stack: "I'm working with Next.js 14, TypeScript, and Tailwind CSS"
- Project architecture: "This is for a microservice using Clean Architecture principles"
- Existing patterns: "We follow the repository pattern for data access"
- Constraints: "The solution needs to work in environments without browser APIs"
For example:
"I'm building a React Native app with Expo and need to implement a caching system for API responses. We're using React Query for data fetching, AsyncStorage for persistence, and follow a custom hook pattern for shared logic. Please create a hook that handles caching API responses with configurable TTL and fallback to cached data when offline."
This detailed context enables the AI to generate code that aligns with your specific environment and conventions.
Beyond Code Generation: Strategic Use Cases
While generating code snippets is valuable, AI coding assistants excel in several other areas that can enhance your development workflow:
Code Transformation
Use AI to refactor or transform existing code:
// Prompt:
"Convert this JavaScript class-based component to a functional component with hooks:
class UserProfile extends React.Component {
constructor(props) {
super(props);
this.state = { user: null, loading: true };
}
componentDidMount() {
fetchUser(this.props.userId).then(user => {
this.setState({ user, loading: false });
});
}
render() {
if (this.state.loading) return <Loading />;
return <div>{this.state.user.name}</div>;
}
}"
Documentation Generation
Create documentation for existing code:
// Prompt:
"Generate JSDoc comments for this function:
function processTransactions(transactions, accountId, options = {}) {
const account = getAccount(accountId);
if (!account) throw new Error('Account not found');
const { skipValidation = false, includePending = true } = options;
let filteredTransactions = transactions.filter(t => t.accountId === accountId);
if (!includePending) {
filteredTransactions = filteredTransactions.filter(t => t.status !== 'pending');
}
if (!skipValidation) {
validateTransactions(filteredTransactions);
}
return calculateBalance(account, filteredTransactions);
}"
Testing Assistance
Generate test cases for your code:
// Prompt:
"Write Jest tests for this authentication utility function:
export function validatePassword(password) {
if (password.length < 8) return { valid: false, reason: 'TOO_SHORT' };
if (!/[A-Z]/.test(password)) return { valid: false, reason: 'NO_UPPERCASE' };
if (!/[a-z]/.test(password)) return { valid: false, reason: 'NO_LOWERCASE' };
if (!/[0-9]/.test(password)) return { valid: false, reason: 'NO_NUMBER' };
return { valid: true };
}"
Handling AI Limitations Gracefully
Despite their capabilities, AI coding assistants have limitations. Understanding these constraints helps set realistic expectations and develop strategies to work around them.
Common limitations include:
- Knowledge cutoffs: AI may not be familiar with the latest framework versions or APIs
- Hallucinations: AI might confidently suggest non-existent functions or methods
- Context windows: There's a limit to how much code or conversation the AI can consider at once
When facing these limitations, try:
- Breaking complex problems into smaller, manageable chunks
- Providing reference documentation links for newer technologies
- Verifying generated code against official documentation
- Using the AI to explain the approach, then implementing it yourself
Conclusion
Effective prompting is the bridge between your development needs and AI's capabilities. By providing clear context, embracing iterative refinement, and understanding both the strengths and limitations of AI coding assistants, you can transform these tools from simple code generators into powerful collaborative partners.
The most successful developers aren't those who rely entirely on AI, nor those who reject it outright, but those who develop the communication skills to work alongside it effectively. As you refine your prompting techniques, you'll find that the quality of your AI collaborations—and your resulting code—improves dramatically.
The future of programming isn't human OR machine—it's human AND machine, working in harmony through the art of effective communication.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.