Rust in AI: The New Frontier for Performance-Critical Machine Learning
2025-04-06
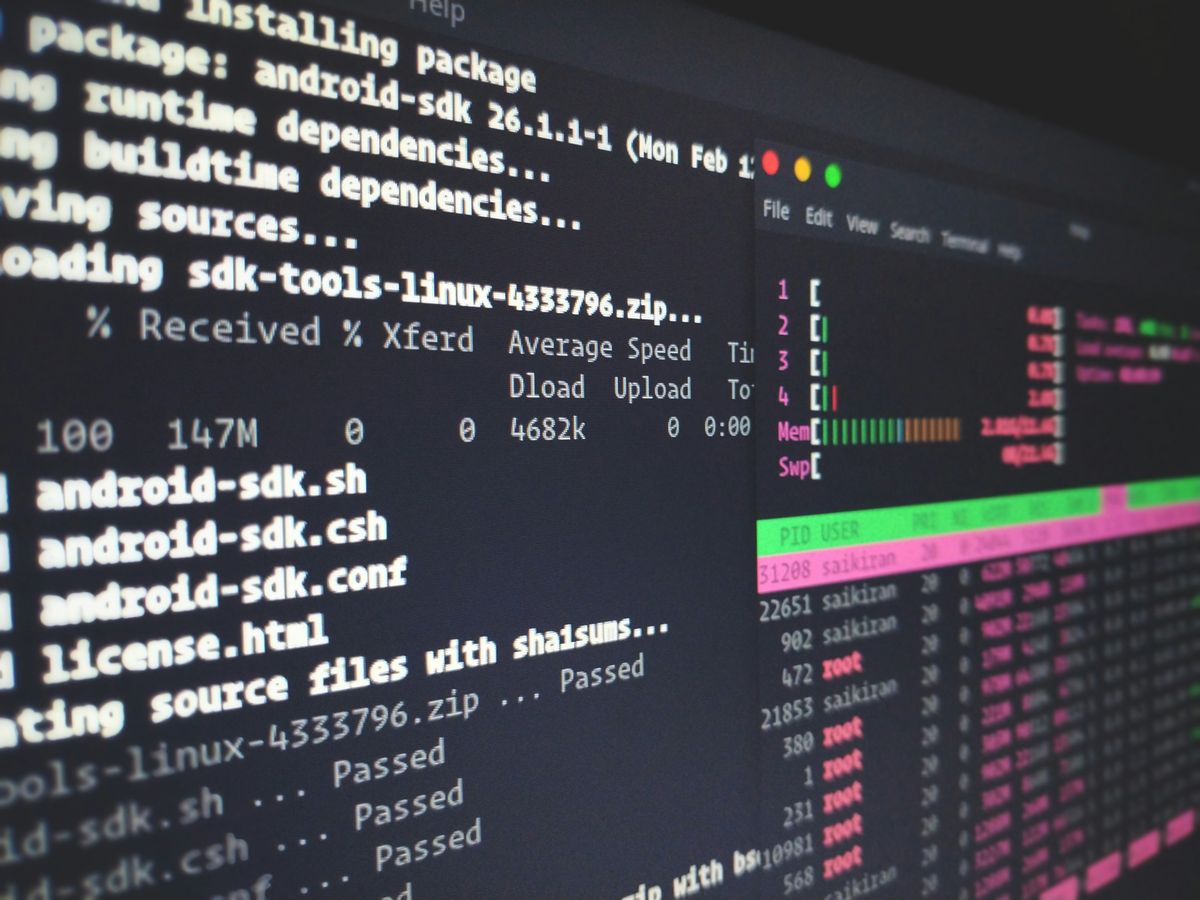
The AI landscape is evolving at breakneck speed, with frameworks and models growing increasingly complex. Behind the scenes, a significant shift is occurring in the infrastructure that powers these systems. Rust, a language designed for performance, reliability, and memory safety, is steadily making inroads into AI development—challenging Python's dominance in specific, performance-critical domains. This transition isn't merely about language preference; it represents a fundamental rethinking of how we build AI systems that can scale efficiently while maintaining safety guarantees.
The Performance Imperative in Modern AI
As AI models grow larger and datasets expand into the petabyte range, the computational demands have reached unprecedented levels. Python, while excellent for research and prototyping, brings significant performance overhead that becomes increasingly problematic at scale.
Rust offers near-C performance without sacrificing memory safety or modern language features. This performance advantage becomes particularly evident in inference engines, data preprocessing pipelines, and embedded AI applications where every millisecond counts.
Consider this comparison of a simple vector operation in Python versus Rust:
# Python with NumPy
import numpy as np
import time
def vector_add(a, b, iterations=1000000):
start = time.time()
for _ in range(iterations):
c = a + b
end = time.time()
return end - start
a = np.array([1.0, 2.0, 3.0])
b = np.array([4.0, 5.0, 6.0])
print(f"Time: {vector_add(a, b):.4f} seconds")
// Rust equivalent
use std::time::Instant;
fn vector_add(iterations: usize) -> f64 {
let a = vec![1.0, 2.0, 3.0];
let b = vec![4.0, 5.0, 6.0];
let start = Instant::now();
for _ in 0..iterations {
let c: Vec<f64> = a.iter().zip(b.iter())
.map(|(&x, &y)| x + y)
.collect();
}
start.elapsed().as_secs_f64()
}
fn main() {
println!("Time: {:.4} seconds", vector_add(1000000));
}
While this is a simplified example, real-world benchmarks consistently show Rust outperforming Python by significant margins for computationally intensive tasks.
Memory Safety Without Compromise
One of AI's often-overlooked challenges is ensuring system reliability as applications scale. Memory leaks, data races, and undefined behavior can cause catastrophic failures in production AI systems—particularly problematic when these systems make critical decisions.
Rust's ownership model and borrow checker eliminate entire classes of bugs at compile time, providing guarantees that are impossible in languages like C++ or Python. This becomes particularly valuable in:
- Distributed training systems where race conditions can corrupt model weights
- Embedded AI applications where memory constraints are tight
- Mission-critical AI systems where failures have significant consequences
The language's "zero-cost abstractions" philosophy means developers don't have to choose between safety and performance—a crucial advantage for AI systems that need both.
The Ecosystem Evolution
For years, Rust's adoption in AI was hindered by a lack of mature libraries compared to Python's rich ecosystem. That gap is closing rapidly with projects like:
- Burn - A dynamic neural network framework written in pure Rust
- Linfa - A Rust-based machine learning framework inspired by scikit-learn
- Tract - A Rust implementation of neural network inference
- Candle - A minimalist ML framework with GPU support
- PyTorch Rust bindings - Allowing seamless integration with the popular framework
These libraries are enabling developers to build hybrid systems that leverage Python's ease of use for research and experimentation while deploying Rust for performance-critical components.
Real-World Applications
The transition to Rust for AI isn't merely theoretical. Several notable applications demonstrate its practical benefits:
Inference Optimization
Hugging Face's tokenizers library, rewritten in Rust, achieves up to 100x speedup compared to its Python predecessor. This performance gain is critical for production systems where tokenization can become a bottleneck.
use tokenizers::Tokenizer;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let tokenizer = Tokenizer::from_pretrained("bert-base-uncased", None)?;
let encoding = tokenizer.encode("Hello, world!", false)?;
println!("Tokens: {:?}", encoding.get_tokens());
Ok(())
}
Embedded AI
Companies building AI capabilities for resource-constrained devices are increasingly turning to Rust. Its predictable performance, minimal runtime, and fine-grained memory control make it ideal for edge AI applications where Python's interpreter and garbage collection would be prohibitive.
Data Processing Pipelines
Data preprocessing often represents the most time-consuming part of AI workflows. Rust-based data processing tools like Polars (a DataFrame library) demonstrate order-of-magnitude performance improvements over pandas for large-scale data manipulation tasks.
Bridging the Gap: Rust and Python Interoperability
The transition to Rust doesn't require abandoning Python entirely. The PyO3 project enables seamless integration between the two languages, allowing developers to gradually migrate performance-critical components to Rust while maintaining compatibility with Python-based workflows.
This hybrid approach is gaining traction in production environments:
use pyo3::prelude::*;
use pyo3::wrap_pyfunction;
#[pyfunction]
fn fast_vector_operation(a: Vec<f64>, b: Vec<f64>) -> PyResult<Vec<f64>> {
let result = a.iter().zip(b.iter())
.map(|(&x, &y)| x * y + (x - y).abs())
.collect();
Ok(result)
}
#[pymodule]
fn rust_ml(_py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(fast_vector_operation, m)?)?;
Ok(())
}
This code creates a Python-callable Rust function that performs a complex vector operation far faster than pure Python could achieve.
Conclusion
Rust's emergence in the AI ecosystem represents more than just another language option—it signals a maturation of the field. As AI moves from research labs to production environments with strict performance, safety, and reliability requirements, the tools we use must evolve accordingly.
While Python will continue to dominate AI research and prototyping for the foreseeable future, Rust is carving out a crucial role in the performance-critical infrastructure that powers production AI systems. For developers looking to build the next generation of AI applications, gaining fluency in Rust may prove as valuable as understanding the latest neural network architectures.
The future of AI development likely isn't an either/or proposition between Python and Rust, but rather a complementary approach that leverages the strengths of each. As the boundaries between research and production continue to blur, the languages and tools that can effectively bridge this gap will become increasingly essential to the AI developer's toolkit.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.