The Compiler Renaissance: How MLIR is Unifying AI and Traditional Programming
2025-04-05
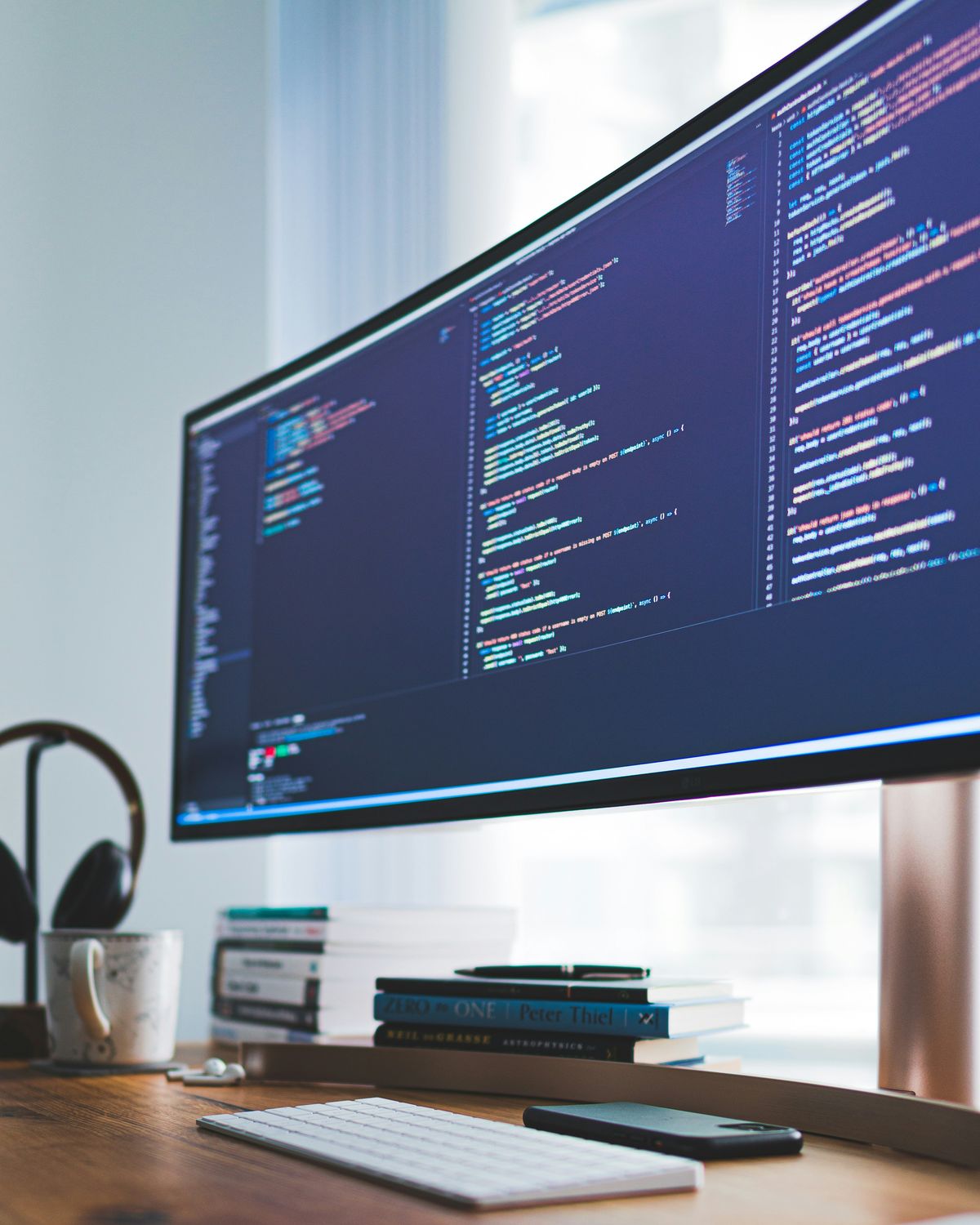
In the divided landscape of computing, AI frameworks and traditional programming languages have long existed in separate domains, with different optimization techniques, toolchains, and communities. But beneath the surface, a quiet revolution is taking place at the compiler level. MLIR (Multi-Level Intermediate Representation) is emerging as a technological bridge that's unifying these worlds, enabling a new generation of performance optimizations and cross-framework compatibility that was previously impossible.
The Compiler Crisis in AI Development
The explosion of AI frameworks has created a fragmentation problem. PyTorch, TensorFlow, JAX, ONNX, and dozens of specialized frameworks each implement their own compiler stacks and optimization pipelines. This fragmentation means that optimizations developed for one framework rarely benefit others, hardware vendors must support multiple targets, and developers face significant barriers when trying to combine techniques from different ecosystems.
Consider this common scenario: a team develops a cutting-edge model in PyTorch for research, but their production environment runs optimized TensorFlow. The conversion process is often manual, error-prone, and loses critical optimizations:
# Research code in PyTorch
class ComplexModel(nn.Module):
def __init__(self):
super().__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3)
self.attention = CustomAttentionBlock(64)
# More layers...
def forward(self, x):
x = self.conv1(x)
x = self.attention(x)
# Complex operations that may not translate well
return x
# Production conversion to TensorFlow often loses optimizations
# and requires significant reworking
MLIR addresses this fundamental disconnect by providing a common compiler infrastructure that different frameworks can target.
What Makes MLIR Revolutionary
At its core, MLIR is a compiler infrastructure that allows multiple levels of abstraction to coexist within the same framework. Unlike traditional compilers with fixed intermediate representations, MLIR enables domain-specific "dialects" that can represent everything from high-level neural network operations to low-level hardware instructions.
This multi-level approach is particularly powerful for AI workloads, which typically start as high-level tensor operations but need to be progressively lowered to specialized hardware instructions for GPUs, TPUs, or custom accelerators.
The key innovation of MLIR is its dialect system:
// High-level TensorFlow operations
%0 = "tf.Conv2D"(%input, %filter) {strides = [1, 1, 1, 1], padding = "SAME"} : (tensor<1x28x28x1xf32>, tensor<3x3x1x32xf32>) -> tensor<1x28x28x32xf32>
// Can be lowered to linalg operations
%1 = linalg.conv_2d_nhwc_hwcf
{dilations = dense<1> : tensor<2xi64>, strides = dense<1> : tensor<2xi64>}
ins(%input, %filter : tensor<1x28x28x1xf32>, tensor<3x3x1x32xf32>)
outs(%output : tensor<1x28x28x32xf32>) -> tensor<1x28x28x32xf32>
// Eventually reaching LLVM IR or hardware-specific instructions
This unified representation allows optimizations to be shared across frameworks and hardware targets, dramatically reducing duplication of effort.
Breaking Down the AI Framework Silos
The practical impact of MLIR is already being felt across the AI ecosystem. TensorFlow adopted MLIR as its core compiler infrastructure with TFRT (TensorFlow Runtime). PyTorch is integrating with MLIR through its torch-mlir project. Even ONNX, designed for interoperability, is building ONNX-MLIR to improve performance and hardware support.
This convergence means that developers can increasingly mix and match components from different frameworks:
# Future interoperability might look like this
import torch
import tensorflow as tf
from mlir_interop import compile_for_target
# Define model in PyTorch
class MyModel(torch.nn.Module):
# ... model definition ...
# Train in PyTorch
model = MyModel()
train_pytorch(model)
# Compile the model for TensorFlow deployment using MLIR
tf_model = compile_for_target(model, target="tensorflow")
# Deploy in TensorFlow ecosystem
tf.saved_model.save(tf_model, "production_model")
This interoperability reduces the "framework lock-in" that has plagued AI development, allowing teams to choose the best tools for each part of their workflow.
Democratizing Hardware Acceleration
Perhaps the most transformative aspect of MLIR is how it's democratizing access to hardware acceleration. Traditionally, supporting a new AI accelerator required extensive work with each framework. With MLIR, hardware vendors can focus on a single integration point.
This has led to an explosion of support for diverse hardware targets, from established players like NVIDIA and Intel to specialized AI accelerators from startups. The result is that cutting-edge hardware optimizations become available to all frameworks that target MLIR, not just those with the resources for custom integrations.
For developers, this means code like the following becomes increasingly portable across hardware:
# Code written once can be optimized for multiple targets
@tf.function
def process_image(image):
# Complex image processing with neural networks
return result
# Deploy to different hardware through MLIR
cpu_version = tf.lite.TFLiteConverter.from_concrete_function(
process_image.get_concrete_function()).convert()
edge_tpu_version = tf.lite.TFLiteConverter.from_concrete_function(
process_image.get_concrete_function()).convert()
edge_tpu_version = edge_tpu_converter.with_edge_tpu()
# Both versions share optimizations through the MLIR pipeline
Bridging Research and Production
The research-to-production gap has long been a pain point in AI development. Researchers prefer flexible frameworks that prioritize experimentation, while production engineers need optimized, stable deployments. MLIR helps bridge this gap by allowing research code to be progressively optimized and hardened without complete rewrites.
This capability is creating new workflows where research prototypes can evolve into production systems through a series of increasingly optimized representations, rather than through the traditional rewrite approach:
- Researchers develop in high-level frameworks
- MLIR-based tools progressively optimize the model
- Production engineers apply hardware-specific optimizations
- The same core representation flows through the entire process
This continuity preserves algorithmic integrity while enabling the performance optimizations needed for production.
Conclusion
MLIR represents a fundamental shift in how we think about the relationship between AI frameworks and traditional programming. Rather than separate worlds with occasional bridges, we're moving toward a unified compiler ecosystem where optimizations flow freely across framework boundaries.
For developers working at the intersection of AI and traditional programming, MLIR offers a glimpse of a future with fewer artificial barriers. The ability to mix and match components from different frameworks, target diverse hardware without custom code, and smoothly transition from research to production will fundamentally change how we build AI systems.
As MLIR adoption continues to grow, we can expect to see not just performance improvements, but entirely new programming models that blend the best aspects of neural network frameworks with traditional languages. The compiler renaissance is just beginning, and it promises to reshape the landscape of AI development for years to come.
Enjoyed this article?
Subscribe to get notified when we publish more content like this.